How To Delete Def
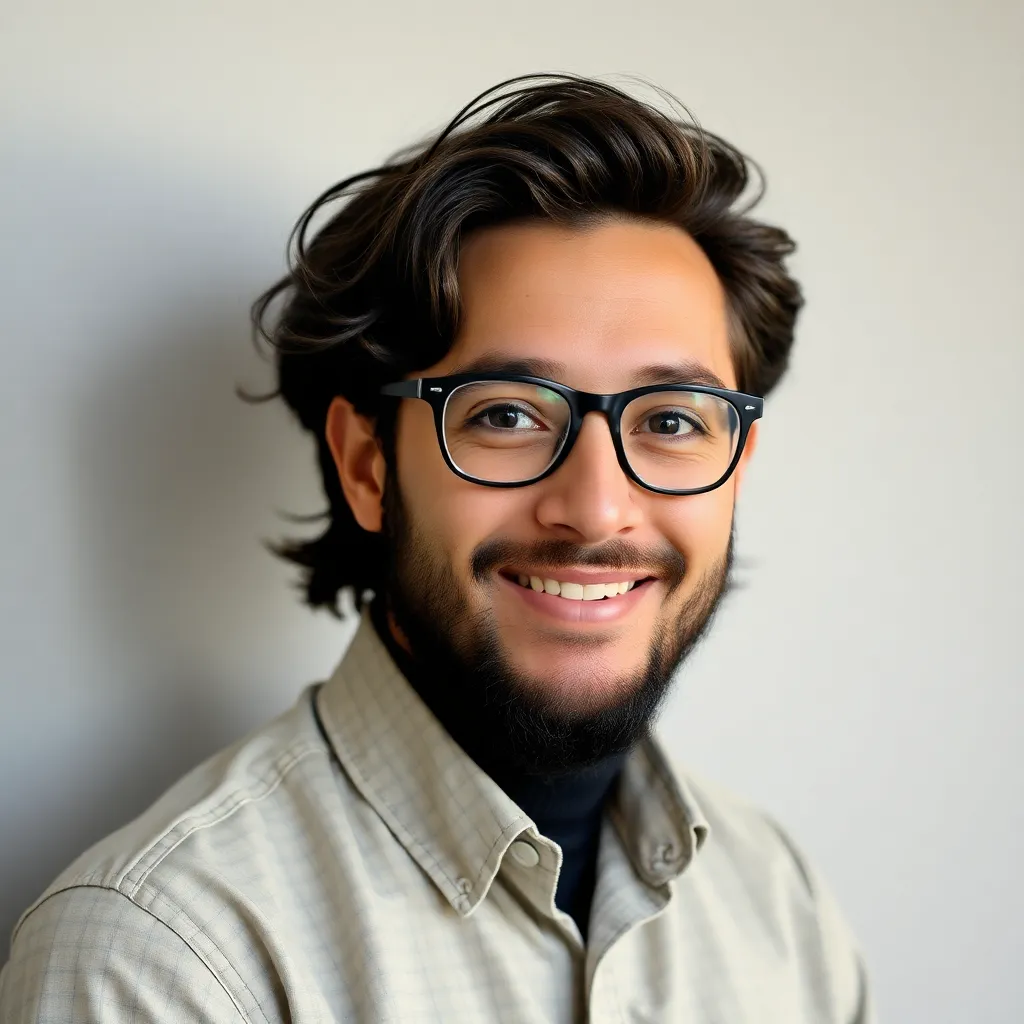
Ronan Farrow
Apr 12, 2025 · 3 min read

Table of Contents
How to Delete a Definition in Python
Python doesn't have a built-in "definition" data type in the same way some other languages might. The term "definition" typically refers to several different things depending on context. To help you effectively delete, we need to clarify what you want to remove. This guide covers the most likely scenarios.
Scenario 1: Deleting a Function Definition
If you're aiming to remove a function's definition from your Python code, the solution is straightforward: simply delete the lines of code that comprise the function.
Example:
Let's say you have a function defined like this:
def my_function(x):
"""This is a sample function."""
return x * 2
To delete it, you would simply remove these lines from your Python file. After deleting and saving the file, the function my_function
will no longer be accessible in your script.
Important Consideration: Ensure you understand the implications of removing a function. If other parts of your code rely on my_function
, removing it will lead to errors when you try to run the script.
Scenario 2: Removing a Variable Definition
Deleting a variable is equally simple. Python manages memory automatically, so once a variable is no longer referenced, it's eligible for garbage collection.
Example:
my_variable = 10
# ... some code ...
del my_variable # Explicitly delete the variable. Not strictly necessary.
After the del
statement, my_variable
is deleted. Again, if other parts of your code use my_variable
, removing it will cause issues. The del
statement isn't always necessary; Python's garbage collection will automatically handle this when the variable is out of scope.
Scenario 3: Removing Dictionary Key-Value Pairs (Definitions in a Dictionary)
If you consider a key-value pair in a dictionary as a "definition," you can remove it using the del
keyword or the pop()
method.
Example:
my_dictionary = {"a": 1, "b": 2, "c": 3}
del my_dictionary["b"] # Removes the key-value pair where key is "b"
print(my_dictionary) # Output: {'a': 1, 'c': 3}
removed_value = my_dictionary.pop("a") # Removes and returns the value associated with "a"
print(my_dictionary) # Output: {'c':3}
print(removed_value) # Output: 1
The pop()
method is beneficial because it also returns the removed value; if you need this value for further processing, it's a handy choice. Using del
on a non-existent key will raise a KeyError
, but pop()
allows you to handle the case where the key might not exist.
Scenario 4: Deleting Class Definitions
Similar to functions, deleting a class definition involves removing the entire class code block from your Python file. This renders the class inaccessible for instantiation and usage. Remember that objects created from the class might still exist in memory unless explicitly deleted (using del
).
Example:
class MyClass:
pass # Class definition
# ... some code using MyClass ...
# To delete, remove the entire class definition.
Best Practices
- Careful Code Review: Before deleting any definition, thoroughly review your code to ensure you understand the consequences.
- Version Control: If working on a substantial project, always use version control (like Git) to easily revert any accidental deletions.
- Testing: After making changes, thoroughly test your code to identify any errors resulting from the deletion.
By understanding these scenarios, you can effectively remove function, variable, and dictionary entry definitions from your Python code. Always remember to back up your work and test thoroughly after making changes.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How To Choose An Egg Donor | Apr 12, 2025 |
How To Clean O2 Sensor With Brake Cleaner | Apr 12, 2025 |
How To Clean A Beehive | Apr 12, 2025 |
How To Clean 6 0 Turbo Without Removing | Apr 12, 2025 |
How To Cook A Kosher Turkey | Apr 12, 2025 |
Latest Posts
-
How To Get A Free Washer And Dryer
Apr 13, 2025
-
How To Get A Fountain Pen Flowing Again
Apr 13, 2025
-
How To Get A Farm Tag In Nc Without
Apr 13, 2025
-
How To Get A Dump Card
Apr 13, 2025
-
How To Get A Domestic Partnership In Illinois
Apr 13, 2025
Thank you for visiting our website which covers about How To Delete Def . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.